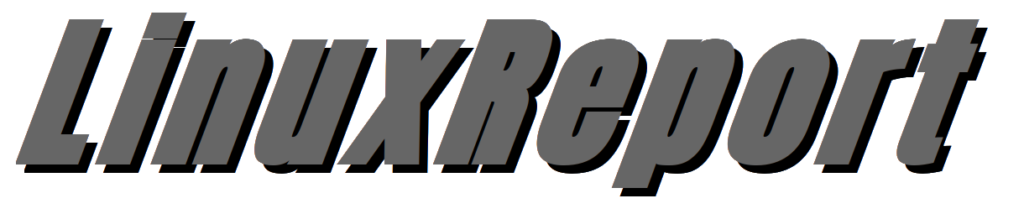
How This Project Made Me a Better Python Developer—And How It Can Help You Learn Flask
When I first set out to build LinuxReport, my goal was simple: create a fast, customizable news aggregator, like DrudgeReport, for the Linux community. It was also a great way to learn Flask and the art of writing efficient web applications. This codebase isn’t just a tool for staying informed, it’s a tutorial for anyone looking to level up their Python skills and dive into real-world Flask development.

One of the reasons I wanted to build it is as a reminder that you can build fast Python applications. My code typically returns the page in milliseconds. And since it’s running on a Linode server with very-high bandwidth, my cheap $5 / month VM can serve 100 requests per second!
Why This Project? Lessons in Problem-Solving
As a FOSS enthusiast, I was tired of juggling a bunch of tabs to track Linux news. I created a simple page with feeds from my favorite sites, lxer.com, LWN.net, Slashdot, Reddit, and more.
- Flask’s Simplicity for Rapid Prototyping:
Flask’s minimalist design let me spin up a working version in hours. Routing with@app.route
, templating with Jinja2, and middleware likeFlask-Mobility
(for mobile detection) turned complex problems into a few lines of code. For beginners, Flask is simple and forgiving—you see results fast, which keeps motivation high. - Concurrency Without Overengineering:
Fetching 10+ RSS feeds sequentially was slow. Switching toconcurrent.futures.ThreadPoolExecutor
taught me how Python handles threading. Suddenly, feeds loaded in parallel, illustrating the benefits of concurrency for I/O-bound tasks—despite Python’s Global Interpreter Lock (GIL). - Caching: The Art of Balancing Speed and Freshness:
Building theFSCache
class (usingFlask-Caching
) allowed me to return pages typically in a few milliseconds, but forced me to think about resource management. How long should a Reddit feed stay cached? These are questions every backend developer faces, and here, I solved them with filesystem-based caching stored in/run
—a skill I’ve reused in other projects.
The flexibility of this codebase shines in its real-world deployments.
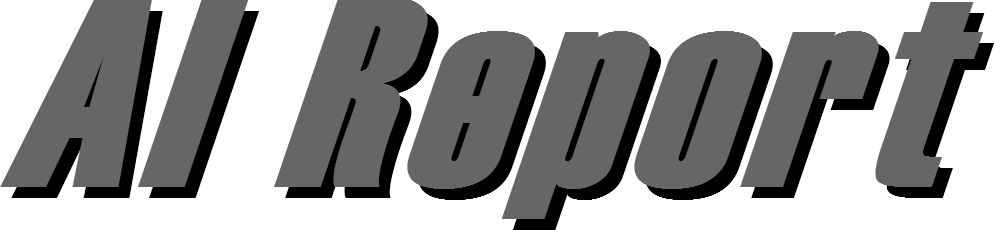
For instance, AIReport aggregates exciting feeds like Reddit’s r/LocalLlama and VentureBeat’s AI section, while
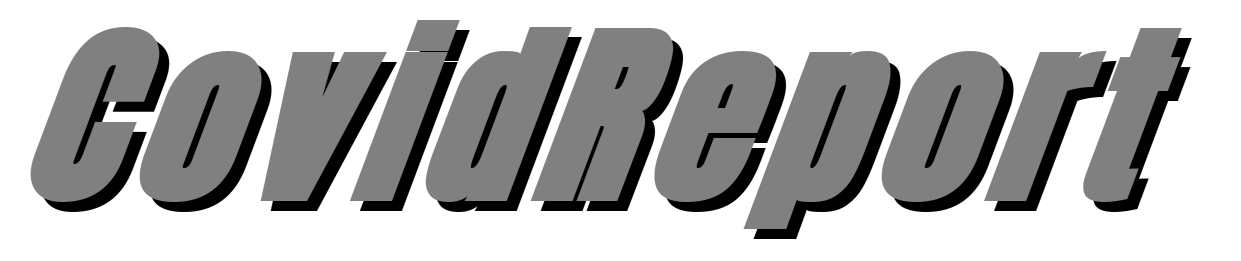
COVIDReport curates critical updates from the CDC, Reddit communities, and YouTube experts like Dr. John Campbell.
Want to track space or other trends? Fork, tweak the feeds, and deploy. The core logic remains untouched, but the output transforms entirely.
Features That Taught Me Advanced Python
Every feature in LinuxReport doubled as a learning opportunity:
- Dynamic Scraping with
AutoScraper
:
Some sites (like Bandcamp) lack RSS. UsingAutoScraper
, I built a system to extract content dynamically. This taught me how to handle edge cases (e.g., missing elements) and the importance of fault tolerance—skills critical for web scraping careers. - Race Conditions and the
FETCHMODE
Flag:
When multiple users triggered feed updates simultaneously, the server buckled. Fixing this with aFETCHMODE
flag (to lock refresh threads) was my introduction to thread safety. It’s a small feature with big lessons in concurrency control. - Cookie-Driven Customization:
Storing user preferences (like the infamous Dark Mode, no underlines, or custom URLs) in cookies seemed simple—until I added versioning (URLS_COOKIE_VERSION
) to handle schema changes. This highlighted the importance of backward compatibility, a must-know for maintaining production apps.
One of my favorite additions is the client-side pagination system. Instead of overwhelming users with 20+ links per feed, entries are split into bite-sized pages (8 items each) with simple “< >” controls. This creates a simple site which lets you explore a rabbit hole.
I had Llama 3.3 70B write the Javascript for this. I never bothered to learn it, I’m more interested in spending time to improve my Python skills.
Llama 3.3 was amazing, the only mistake was the LLM’s initial suggestion to fetch new data dynamically. Instead, I told it to have all entries pre-loaded but hidden via CSS (display: none
), eliminating server roundtrips while keeping the snappy feel. It’s a perfect example of balancing discoverability with performance, handled through vanilla JavaScript’s DOM manipulation.
Final Thoughts: Why I’m Proud of This Project
LinuxReport started as a weekend experiment. Today, it’s a testament to how building something useful can accelerate your learning. I went from Flask novice to confidently designing threaded, cached, and scalable apps—all while solving a problem I cared about.
LLMs are also definitely changing software development and eventually white-collar jobs. I look forward to the day when I can use an LLM inside my favorite Linux apps like LibreOffice.
If you’re a Python developer looking for hands-on experience with Flask or simply want to explore building fast and scalable applications, I invite you to fork LinuxReport, make it your own, and enjoy the journey.